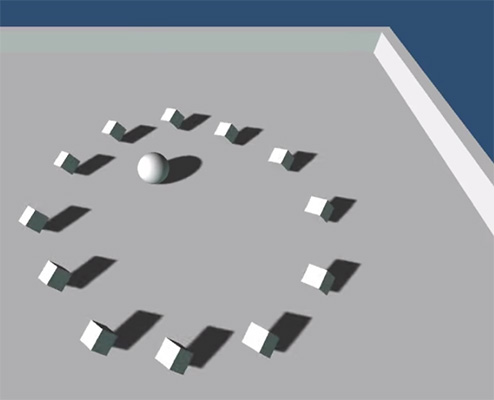
Concepts
Game programming involves a number of concepts which are not typical of normal development. Experienced game developers can probably skip this section, but I'm going to walk through some of the things you need to know in order to make your first game, and then how I learned some of the specifics of developing in Unity3d.
Scene Graph
The scene graph is how objects in your world are arranged. I'm going to add and link to a more thorough explanation of it at some point in the future, but for now just think of how you'd find an empty bottle on a pirate ship: the ship is at some latitude, longitude, and heading on the ocean surface. The bottle is rolling back and forth across the deck, but remains on the ship itself. Everywhere the ship goes, every time it turns, the bottle follows along. The bottle is parented to the ship.
The scene graph captures and represents these relationships, so that we have a consistent and straightforward way to move items around in the scene (and ultimately, on the screen of the display.) It's easier to refer to the bottle's location on the deck of the ship than in the world at large.
Under the hood, we store the positions and rotations of items in mathematical matrices. The reason is that it's the fastest way we know of to actually transform and translate them in order to move them around or figure out where they belong on the screen. It's unlikely that as a modern game developer you'd ever need to interact with them directly, but it's good to know what's going on.
In Unity3D
The scene graph is clearly represented in a hierarchical form on the left side of the screenshot below, and rendered on the right.
Check out the Unity3d manual for more about the hierarchy and scene views.
Game Loop
Most 3d games are generally built around a main loop which performs a fixed set of tasks in order to render each frame. The same things are done again and again until the player wins or loses, and returns to some sort of menu system in order to start a new game. This isn't the place for an in-depth discussion of that loop, but here are some of the key elements:
- Process input from the player: key presses, mouse or joystick position, orientation of a mobile device, etc.
- Update the position of objects in the world
- Render everything to the screen
In Unity3d
Now, one of the main reasons to use a 3d engine—in fact, one way to define what a 3d engine is—is letting the engine take care of steps #1 and #3 for you. Instead of building this loop yourself, in Unity we attach bits of code to various game objects and let them determine where they should move to, what forces should be applied, and so on. We put this code in a function called Update() and trust that the engine will call it once every time it is rendering a new frame.
In fact, there are a number of functions that it will invoke at different times in Unity:
- Start: Called exactly once per object (player, wall, projectile—anything in the scene graph is called a GameObject)
- Update: Called once per frame for any purpose
- FixedUpdate: Called on a different schedule, specifically for physics-related work
- LateUpdate: Called after the two above, letting you make follow-up adjustments. The manual suggests updating a camera position is one use. (There are virtual camera(s) in any scene which determine what the screen will show. They may be panned, zoomed, and so on just like cameras in the real world.)
- Collision Events: Invoked on objects that bump into each other, which you may use to do all sorts of things.
- Gui Events: Triggered when a player hits a button or other interface element.
There are many more, that are all invoked at clearly-specified times and conditions. The ones I mentioned above are just some of the most important ones.
Starting Out
When teaching myself Unity, I began by going through a couple of the tutorials that they had online. Because I'd been building 3d worlds for almost 10 years, I had a fair sense of what to expect; I just needed to learn some details of the interface for the editor, and get used to the programming environment. In particular, I got a great deal out of these two tutorials:
- Roll-a-ball - an excellent, step-by-step, starting point. I did it twice: once with the instructions, and again a few days later from memory.
- Space Shooter - this goes a little further and also proved essential for learning how to work with real "assets" and the like.
In my next post, I'm going to write a little bit about the design of the game I'm building and why it is my goal.